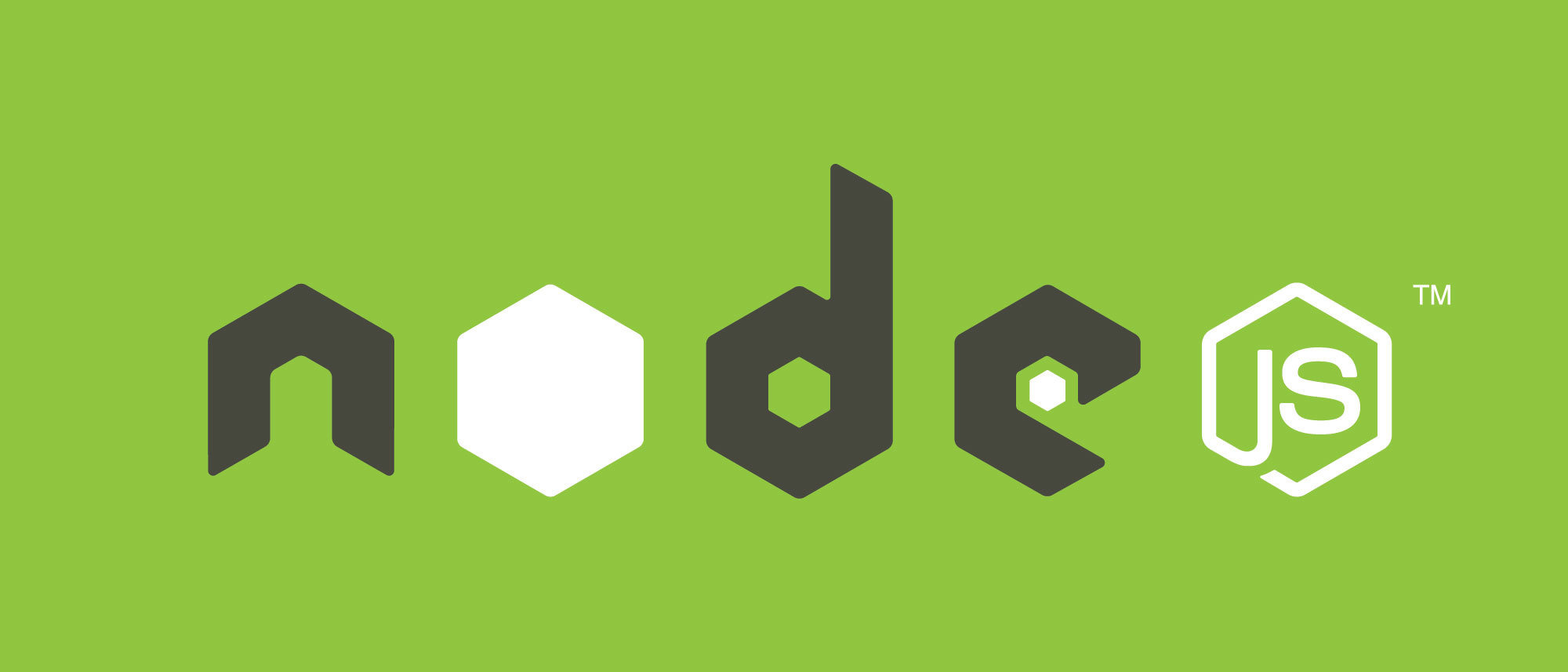
What is the best way to run a NodeJS app on a server? For a long time, I simply used the "screen" command, which had no process monitoring or anything like that. The next attempt was "forever", which now ensures that the process is automatically restarted if it dies.
But the best solution I could find was "pm2". The "Advanced, production process manager for Node.js" offers process monitoring as well as support for load balancing, log file management, git pull, and much more.
pm2 is installed very simply with
> npm install pm2 -g
Our NodeJS application can then be started:
> pm2 start app.js
Most of this has already happened. Pm2 now runs the app and restarts it if necessary.
You can list all processes with
> pm2 list

You can get details about a particular process by entering
> pm2 show appName/appId

And a monitoring of all processes can be displayed like this
> pm2 monit

I was particularly convinced by pm2 if you want to use load balancing. To do this, you only have to start the app with one additional parameter:
> pm2 start app.js -i 4
With "-i 4" pm2 is instructed to start 4 worker instances of the application. The number of workers can be changed at any time with
> pm2 scale appName 2
(in which case the number of workers is reduced to 2).
The nice thing about this is that pm2 takes care of the clustering. You don't have to use a load balancing function in the NodeJS app, the so-called "clustering" is completely done by pm2.
This means that this code becomes superfluous:
var cluster = require('cluster');
var http = require('http');
var os = require('os');
var numCPUs = os.cpus().length;
if (cluster.isMaster) {
// Master:
// Let's fork as many workers as you have CPU cores
for (var i = 0; i < numCPUs; ++i) {
cluster.fork();
}
} else {
// Worker:
// Let's spawn a HTTP server
// (Workers can share any TCP connection.
// In this case its a HTTP server)
http.createServer(function(req, res) {
res.writeHead(200);
res.end("hello world");
}).listen(8080);
}
Instead, you can concentrate on the essentials:
var http = require('http');
http.createServer(function(req, res) {
res.writeHead(200);
res.end("hello world");
}).listen(8080);
Leave a comment now